Golang for Devops and Cloud Engineers
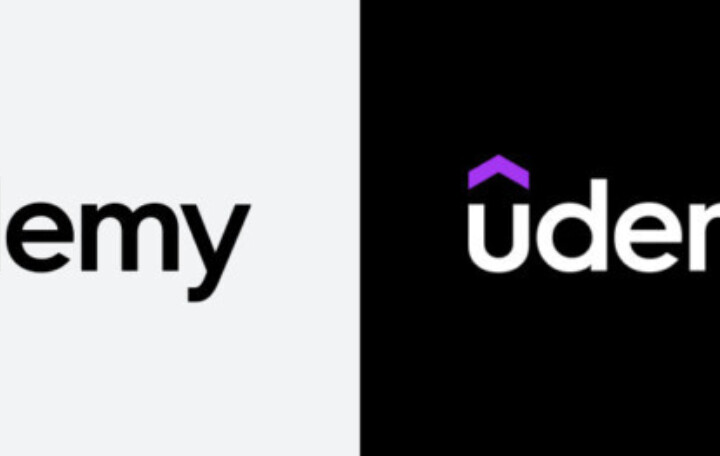
Golang for Devops and Cloud Engineers is an excellent course from Edward Viaene I completed recently (certificate here). The course covered about 20 hours of videos, and took about 45 hours to complete including the 'assignments' (actual programming).
I have specific interest in Golang since it results in static binaries for multiple platforms, it can be run without any dependencies and has excellent concurrency support. And I like how error handling is so much 'part' of Golang, as opposed to 'exceptions' thrown in other languages (dependent upon the framework). In fact, many of the lines of code you'll write is about 'is there an error and if yes what will I return or do with it'. That sounds tiresome, but really it is about writing stable applications.
So my background is that I have already written some Golang applications, functions, that I run in AWS Lambda, and some command line programs for my own use to make devops life easier.
The course started off slowly with some Golang concepts and techniques worth knowing (such building and packaging, channels and asynchronous 'go' routines'), but then quickly took off with an in depth explanation of how to implement some real-world use cases.
The use cases were accompanied by assignments or challenges, that mostly followed a test driven approach: the instructor first explained the task / use cases at hand, and then provided the unit tests and basic skeleton to implement the use case and features.
The student was then invited to complete the task by writing the code until the requirements in the unit tests were met. Then, a possible explanation of the implementation was offered (ofc I deviated from this at some points). This ultimately led to complete example applications of various interests:
- Accessing and managing AWS and Azure services from within Golang
- Controlling Kubernetes with Golang
- Creating your own SSH server
- And OIDC authorization server and client
- Writing a TLS and mTLS server in Go
- Writing a DNS resolver in Go
The AWS and Azure projects (authenticate, create bucket, create instance etc) were quite obvious to me since I have experience with the AWS Api from other programming languages. But then, it was nice to see the differences in approach between AWS and Azure.
The Kubernetes project showed how to deploy to pods on Kubernetes programmatically. Included in this project was how to write (and test!) Github web hooks in Go to create a simple deployment pipeline for your local MiniKube cluster.
Creating an SSH server was a project that interested me. It showed how to create an integrated SSH server in your application. And then execute commands in that app, using a terminal or by supplying them with the SSH command. I see how this can be useful, i.e. you could send files [scp] or commands [ssh] directly to your application using a secure channel.
Also, the internals about an SSH server were quite interesting.
The OIDC assignment I found most interesting. It covered how to write an authorization server with OpenId connect. Not only was the assignment helpful in getting insight in the OIDC process, the instructor also showed how to connect this server to AWS IAM and to grant the user supplied by the Golang authorization server permissions to AWS resources without creating IAM users. And, to use the authorization server to authorize users in Jenkins. Really very interesting, and fun to complete. I pushed my code to this GitLab repository. (Mind this is not production code, a would do a lot of things differently for production code).
Then, TLS in go, not only showed how to write a TLS (https) server in Go, which is quite obvious, but also how to create your own CA and server/client certificates. The final use case was to write a mutual (two way) TLS server and client, using server and client certificates created within the Go application itself. This allows secure and encrypted communication between microservices for example (though there are more purposes ofc).
As a bonus it was shown how easy it is to create a TLS server in Golang using LetsEncrypt certificates: just a few lines and you have your zero config https server running. Wow.
Writing a DNS resolver was of mediocre interest, it's not something that one would do everyday when you are a devops engineer I guess. Nevertheless, it showed how to establish communication with a Golang application using UDP packets, and covered the process of DNS resolving (which is a bit more complicated than suspected).